Micro:bit
Regarding tasks related to a Micro:bit, it is advantageous to opt for the response to the code in the form of sound or movement. For instance, you can connect a propeller to the Micro:bit or program a potential burglar alarm with sound as feedback.
Microsoft MakeCode is a free online learn-to-code platform. It has built-in support for screen readers (such as JAWS), allowing users to write and read text-based code in JavaScript or Python.
In this task the students are supposed to experiment with pre-made code for a “Burglar alarm” and receive audio feedback. The Micro:bit has a built-in function that can detect light levels, which we can use to create an alarm. The task is to build an alarm that can be placed in a dark environment and is triggered when this environment becomes bright, for example, when a cabinet door is opened.
The teacher has pre-programmed blocks and switched to Python code in advance. Create a Word document with tasks for the students.
Have students test and manipulate the code.
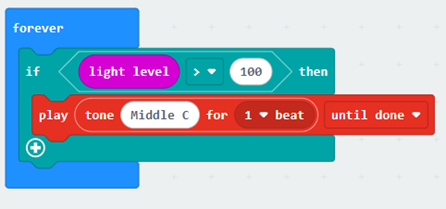
def on_forever():
if input.light_level() > 100:
music.play(music.tone_playable(262,music.beat(BeatFraction.WHOLE)), music.PlaybackMode.UNTIL_DONE)
basic.forever(on_forever)
In this task the students are supposed to experiment with pre-made code for “Jingle Bells” and receive audio feedback. The teacher prepares tasks with code in a Word document. The student pastes the finished code into the MakeCode editor, listens to the result, and can then adjust the code in the editor to test the result again.
Preparations: The teacher has pre-programmed blocks and switched to Python code in advance. Create a Word document with tasks for the students.
For example, the first part of Jingle Bells:
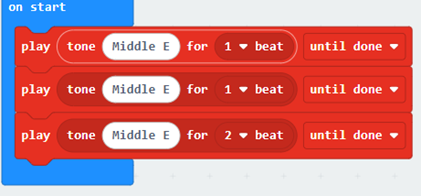
music.play(music.tone_playable(330, music.beat(BeatFraction.WHOLE)), music.PlaybackMode.UNTIL_DONE)
music.play(music.tone_playable(330, music.beat(BeatFraction.WHOLE)), music.PlaybackMode.UNTIL_DONE)
music.play(music.tone_playable(330, music.beat(BeatFraction.DOUBLE)), music.PlaybackMode.UNTIL_DONE)
def on_forever():
pass
basic.forever(on_forever)
Examples of Tasks- Copy the code for the first part of Jingle Bells into MakeCode. (Re-delete any text in the editor field by selecting all with ctrl + a, then press delete). Play the beginning of the song using ctrl + enter.
- How can the first part be played faster? Try changing the tempo and play it using ctrl + enter.
Different tempos:
Whole
Half
Quarter
Eighth
Sixteenth - Repeat the first part. Copy the following code into MakeCode. To delete what is already in MakeCode, select all with ctrl + a, then delete.
How has the code changed? Feel free to manipulate the code further and see what happens when it is played.
for index in range(2): music.play(music.tone_playable(330, music.beat(BeatFraction.WHOLE)), music.PlaybackMode.UNTIL_DONE) music.play(music.tone_playable(330, music.beat(BeatFraction.WHOLE)), music.PlaybackMode.UNTIL_DONE) music.play(music.tone_playable(330, music.beat(BeatFraction.DOUBLE)), music.PlaybackMode.UNTIL_DONE) def on_forever(): pass basic.forever(on_forever)
Block programming - Can you program the next part of the song?
The notes you will need:
C: 262
D: 294
E: 330
G: 392
Programming Mathematics
A good way to get started is to choose a simple task from the math book and ask the students how they could solve it using programming. This also allows the student to link existing knowledge in working memory with new information related to programming. In this example the code is written in Python in Visual studio code.
Carol lives 4.2 km from school, and Billy lives 3.75 km from school. How far is their combined distance to school? How can we solve the problem using programming? Display on the board:
Solution 1:
print(4.2 + 3.75)
The answer is displayed: 7.95We increase the complexity by introducing a variable.
Solution 2:
Carol = 4.2
Billy = 3.75
sum = Carol + Billy
print(sum)
To clarify what the answer means, formulate the last line to:
print("Together, they have ",sum," km to school")
When programming, we often want to ask questions and utilize the program for multiple examples. Use the same example and modify it.
Carol = input("How far does Carol live from school (km)?")
Billy = input("How far does Billy live from school (km)?")
sum = Carol + Billy
print("Together, they have ",sum," km to school")
Let us test! The answer is wrong! That was the point. We utilize the hypercorrection effect, which means that when someone is confident about what the answer should be and it is not, they are more likely to learn something new. We need to let Python know that the information we input is a number or a decimal, not text. We add “float” before input.
Carol = float(input("How far does Carol live from school (km)?"))
Billy = float(input("How far does Billy live from school (km)?"))
sum = Carol + Billy
print("Together, they have ",sum," km to school")
Now we can use this program as a tool in other programming tasks.
The exercise is designed to illustrate the utilization of a Python program in verifying whether a given integer is a prime number. The idea is to solve this with a loop that checks either all numbers up to the entered number or a suitable subset. The level of difficulty can be adjusted through various instructions or clues:
- Present segments of the code that address the problem.
- Enhance efficiency by validating the solution with a reduced set of numbers.
- Instead of confirming the primality of a single number, consider assessing all numbers within a designated range, such as up to 1000.
- Ask the user to enter a prime number.
- Convert the input to an integer.
- Assume that the integer is a prime number (set a variable to True).
- For each number from 2 to the entered number, do the following:
If the entered number can be divided evenly by the current number, set the variable to False.
If not, move on to the next number. - Print whether the number was a prime or not.
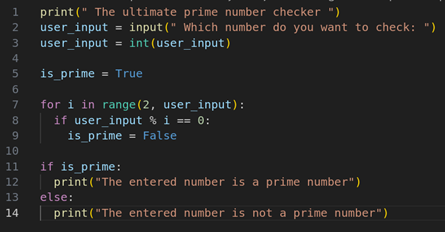
print(" The ultimate prime number checker ")
user_input = input(" Which number do you want to check: ")
user_input = int(user_input)
is_prime = True
for i in range(2, user_input):
if user_input % i == 0:
is_prime = False
if is_prime:
print("The entered number is a prime number")
else:
print("The entered number is not a prime number")
Preparations for task 1: Copy instructions and patterns (visual and tactile)
Preparations for task 2: Code blocks (visual and tactile bricks). Choose a programming environment and a text-based programming language.
Task 1:
- Write an algorithm that displays the number of objects in the two images below.
- Write a formula that displays the number of objects (balls, sticks) in the two images below.


Use the following code blocks:
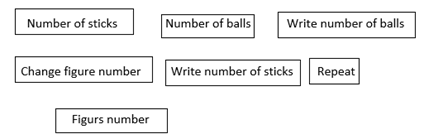