Jump to:
Text-based programming can be a very interesting topic for braille readers. Mainly because a bit of knowledge about programming can enable them to make calculations more efficient.
Programming Environments
There are many different programming environments that schools can use in their teaching regarding computational thinking, and some are more accessible than others. Examples of accessible environments are Excel and Visual Studio Code (VS Code), paired with the Python programming language. Be aware that braille readers employ distinct strategies compared to sighted students when navigating in the programming environment. While a screen reader facilitates accessibility in a digital setting, a braille reader must proficiently navigate using shortcuts. Once these skills are mastered, the braille reader can better concentrate on the computational thinking aspects of the task. Collaboration with ICT professionals may be essential in this regard.
In some cases, for example when a code editor is not accessible, the braille reader may need to compose the code in a Word document, requiring someone else to transfer the text to the code editor. Additionally, attention may be necessary to ensure proper code indentation.
When copying Python-code from a text editor into a code editor it is important to preserve the original indentation and copy from the beginning of the line. In a math book the code might be prefixed with line numbers that are not part of the code, like in the code below, and these numbers and the one following extra space have to be removed before the code can run.
1 def primesLessThan(number):
2 primes = []
3 for i in range(2, number):
4 for prime in primes:
5 if i % prime == 0:
6 break
7 else:
8 primes.append(i)
9 return primes
10
11 print(primesLessThan(30))
The line numbers can be removed manually, but if there are many lines it is much faster to do it with advanced search/replace using regular expressions (regex). In VS Code the search/replace box can be opened with Ctrl + h, and then regex can be toggled on/off with Alt + r. Regex might be too difficult for some, but it can save a lot of time for those who learn it. The regex to find the line numbers looks like this: ^\d+ ?
Explanation:
^ indicates the beginning of a line. It matches the starting position of any line.
\d indicates any digit (0-9).
+ indicates one or more occurrences of the preceding element, which in this case is a digit. If there is more than one digit, it will match all of them.
After + there is a space, and the ? after the space means that it is optional. Put another way, ? indicates zero or one of the preceding element. This is necessary for getting a match for line number 10, which contains no code.
The replace box is empty since we are not replacing the line numbers and the following space with anything. We can use Ctrl-Alt-Enter to execute the search/replace. Now the code can be run.
Overview of the Code
Text codes are typically formatted with visual cues such as brackets and indentations to assist sighted readers in accurate interpretation. The visual layout aids sighted students in quickly grasping the code as a whole, offering an immediate overview.
It is crucial to be aware that this process differs for a braille reader. To read a completed code, the braille reader must start from the first braille cell. As all braille cells are of the same size and positioned on the same line, the reader cannot rely on the visual layout of the expression. The absence of visual cues makes interpretation challenging, requiring the braille reader to sequentially construct an understanding of the code. An additional challenge arises from the low redundancy and difficulty in distinguishing braille characters, elevating the risk of misinterpretation. Pay attention to if the student needs to learn new braille characters such as curly brackets, { }.
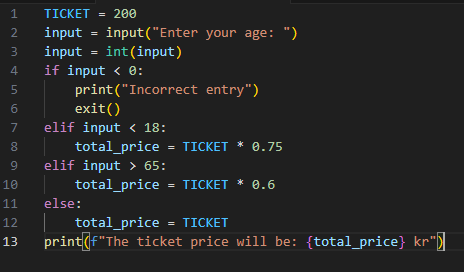
The picture shows a code segment written in Python. The braille reader reads line by line and has to arrow up and down to get an idea of the indentation. There is a lot to keep in mind when the code might have parentheses, curly brackets and loops. As a supplement, to gain a broader overview of the code, it therefore can be valuable for the braille reader to also receive the code in printed braille on paper. Note that braille characters may differ when reading them on paper compared to reading them on a braille display (6- or 8-dot braille).
ICT and Braille Skills
To be able to program, the braille reader must have certain ICT and braille skills. Close collaboration with the professionals of ICT and braille is advised. The ICT skills are mainly about knowing how to navigate through the program, but also about setting the programming environment in such a way that the braille student can work with it properly (settings for the screen reader and speech synthesis that enable the student to navigate and understand the code better). Braille skills are mainly about understanding the structure of a code, understanding how indentation works and knowing all important symbols. Also, braille skills are important to be able to find mistakes in the code. It is important to know that braille is mainly used to understand details of the code and not to read the whole code or to navigate through it very fast.
Uniform Code Standard
When communicating code with others, consistency in writing style is important. This is extra important when a braille reader is communicating the code with the other students, because a non-consistent code can become confusing or difficult to read. A uniform code standard is a set of rules that you use when writing code. When setting these rules there are a couple of things you should keep in mind.
Firstly, it is important to make sure there is enough white space around the signs and text. This makes the text more fluent for the screen reader. Especially when using variables, it is important to put spaces on the right places. If this is done wrong, it is not possible to correctly determine the beginning or end of a variable.
Example:
Variable | Explanation |
---|---|
childrenAndAdolescents | This is one variable, and you can hear that because there is no pauses between the words |
children & Adolescents | These are two variables, and you can hear that because there are pauses between the words |
children&Adolescents | These are two variables, but you cannot hear that because there are no pauses between the words |
A problem with these descriptive variables is that they are very long. Long variables are easier to remember but take a lot of space on the braille display which makes it more difficult to read the rest of the code because the lines will not fit the braille display. This will always be a compromise that the braille reader or teacher has to make. On the one hand variables should not be too long, but on the other hand they should be descriptive, correct and consistent. One could also compromise by leaving white spaces around the variables and operators. How one compromises depends on the preference of the braille reader.
In variables it is advised to start each new word with a capital. This reduces unclarity with speech synthesis for the braille reader.
Example:
NumberOfChildren + NumberOfAdolescents = TotalNumberOfPeople
To Choose Exercises
One way to introduce students to text programming is by providing them with pre-written code to modify. This approach saves time for braille readers and is suitable for collaborative learning. The method is also appealing as it enables the teacher to maintain the level of mathematics at a high standard, surpassing what students might achieve by writing entirely new code on their own.
However, a potential challenge is that the provided code might be too advanced for a student at the beginning of their programming learning journey. As it is more challenging for braille readers to read code and have an overview of the text in text programming, it is especially important to start with simple text strings and then increase the complexity. This approach, to develop the code in small steps can enhance students’ comprehension and, therefore, may serve as a noteworthy example of reverse adaptation.
Even if the objective is to employ computational thinking solution strategies in diverse mathematical tasks it can initially be beneficial to choose outputs that engage senses beyond sight and do not rely on textual interpretation. This may involve using sound reproduction or touch, such as identifying changes in motion for example with a propeller. Designing tasks with variety and where multiple senses are used is valuable for all students. Identifying tasks suitable for collaborative reasoning also enhances the inclusive learning environment.